CRUD API with MySQL and Django Rest Framework with JWT Authentication
Python
CRUD API with MySQL and Django Rest Framework with JWT Authentication
Introduction
In modern web applications, secure authentication is a critical aspect to protect user data and ensure the integrity of the system. JSON Web Tokens (JWT) have become a popular method for implementing stateless authentication in web APIs. In this blog post, we will explore the concept of JWT authentication, why it is used, and how to integrate it into an existing Django REST project.
GitHub Repository
Before we begin, make sure you have a Django REST project set up. This is a continuation from our previous post on Python Django CRUD API with MySQL and Django Rest Framework. You can find the complete code for the project on GitHub: GitHub Repository
What are JSON Web Tokens (JWT)?
JSON Web Tokens (JWT) are an open standard (RFC 7519) for securely transmitting information between parties as a JSON object. A JWT consists of three parts: a header, a payload, and a signature. The header contains information about the type of token and the cryptographic algorithm used to sign it. The payload contains claims or statements about the authenticated user. The signature is generated by combining the header, payload, and a secret key, ensuring the integrity of the token.
Why use JWT Authentication?
JWT authentication offers several benefits for web applications:
Stateless: Unlike traditional session-based authentication, JWTs are stateless. The server does not need to store session information, reducing the server-side overhead and enabling scalability in distributed systems.
Secure: JWTs can be signed using a secret key or a public/private key pair, providing a mechanism for verifying the authenticity of the token. This ensures that the token has not been tampered with and comes from a trusted source.
Cross-Domain Compatibility: JWTs can be easily transmitted over HTTP headers or URL parameters, making them compatible with different client applications, including web, mobile, and desktop.
Extensibility: JWTs allow including custom claims in the payload, enabling the transfer of additional user-specific data along with the authentication information.
Adding JWT Authentication to a Django REST Project
To add JWT authentication to your existing Django REST project, follow these steps:
Step 1: Install Required Packages
Ensure that the djangorestframework_simplejwt
package is installed in your Django project. You can install it using pip
:
pip install djangorestframework_simplejwt
Step 2: Update Settings.py
In your project's settings.py
file, make the following additions:
from datetime import timedelta INSTALLED_APPS = [ # Other installed apps... 'rest_framework', 'rest_framework_simplejwt', ] REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': [ 'rest_framework_simplejwt.authentication.JWTAuthentication', ], } SIMPLE_JWT = { 'ACCESS_TOKEN_LIFETIME': timedelta(minutes=5), 'REFRESH_TOKEN_LIFETIME': timedelta(days=1), }
In the code snippet above, we added 'rest_framework'
and 'rest_framework_simplejwt'
to the INSTALLED_APPS
list. We also configured the DEFAULT_AUTHENTICATION_CLASSES
to include JWTAuthentication
from rest_framework_simplejwt
. Additionally, we set the expiration times for the access token and refresh token using the ACCESS_TOKEN_LIFETIME
and REFRESH_TOKEN_LIFETIME
settings.
Step 3: Define URL Routes
In your project's urls.py
file, add the following URL patterns for token-based authentication:
from rest_framework_simplejwt import views as jwt_views urlpatterns = [ # Other URL patterns... path('auth/token/', jwt_views.TokenObtainPairView.as_view(), name='token_obtain_pair'), path('auth/token/refresh/', jwt_views.TokenRefreshView.as_view(), name='token_refresh'), ]
The code above adds two URL patterns for token-based authentication. The TokenObtainPairView
is responsible for generating a new access token and refresh token pair when provided with valid credentials. The TokenRefreshView
is used to obtain a new access token using a valid refresh token.
Step 4: Adding a User(superuser)
Run the following command in your terminal to create a superuser and access the admin interface:
python manage.py createsuperuser
Follow the prompts to create a superuser account with a username and password. Then, start your Django development server (python manage.py runserver
) and visit http://127.0.0.1:8000/admin/
to verify that the user is listed in the Django admin interface.
Request and Response Examples
Let's take a look at the request and response examples for the newly added authentication endpoints.
Request: Generate Access Token and Refresh Token
- Method: POST
- URL:
http://your-domain/auth/token/
- Body:
{ "username": "admin", "password": "admin" }
- Response:
{ "access": "your-access-token", "refresh": "your-refresh-token" }
Request: Refresh Access Token
- Method: POST
- URL:
http://your-domain/auth/token/refresh/
- Body:
{ "refresh": "your-refresh-token" }
- Response:
{ "access": "your-new-access-token" }
Conclusion
In this blog post, we discussed the concept of JSON Web Tokens (JWT) and why they are used for authentication in web applications. We explored the benefits of using JWT authentication and how to integrate it into an existing Django REST project. By following the steps outlined above, you can enhance the security and scalability of your Django REST API. Feel free to check out the complete code on GitHub to see JWT authentication in action.
Remember to handle the authentication endpoints securely and protect your secret keys and tokens. JWT authentication provides a robust mechanism for securing your web APIs and ensuring a seamless user experience across different client applications. Github Link: CRUD API with MySQL and Django Rest Framework with JWT Authentication
CRUD API with MySQL and Django Rest Framework with JWT Authentication

June 27, 2023
Comments
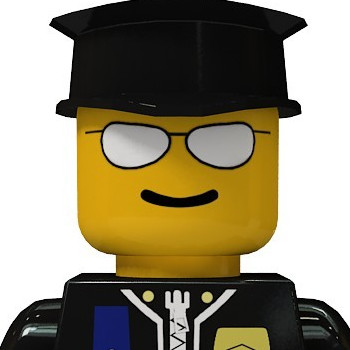
Good job Kevin!