Spring Boot Auto Configuration and Creating Custom Auto Configuration with @Conditional
Java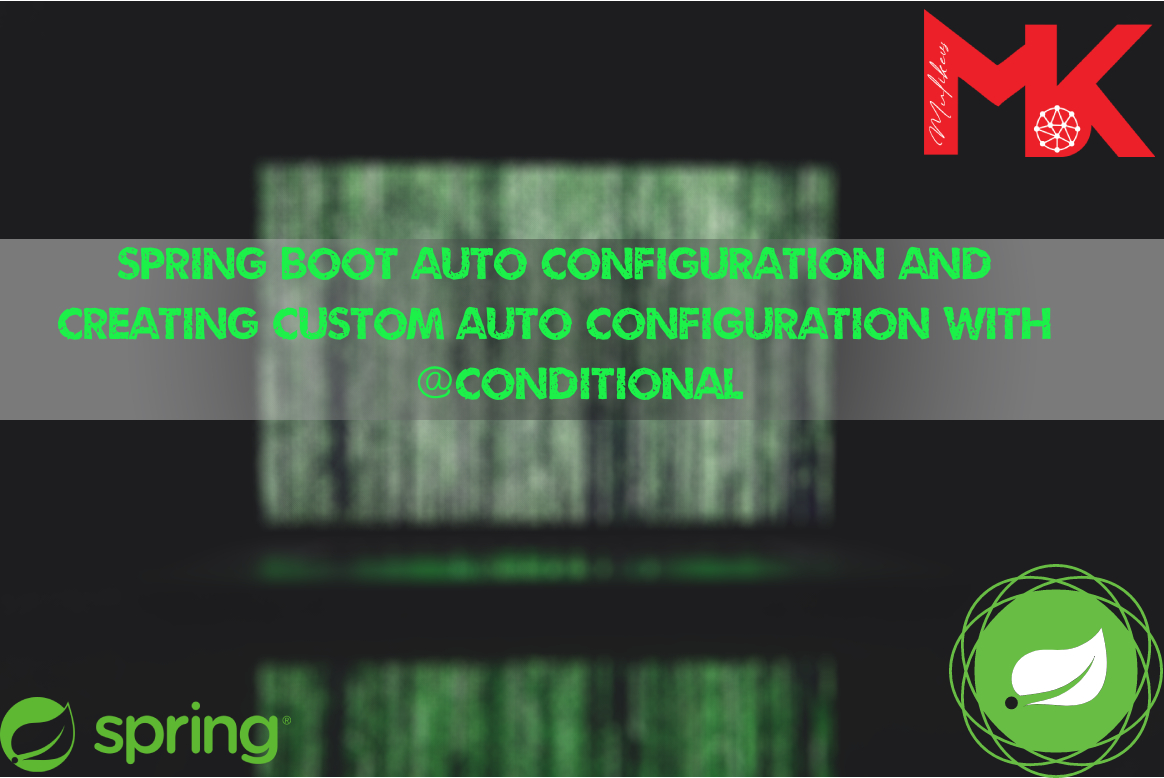
In the world of software development, building applications can often involve a lot of setup and configuration. Spring Boot, a popular framework, comes to the rescue with its magical Auto Configuration feature. If you're new to Spring Boot, don't worry - we're here to break down this concept in simple terms, show you real-world examples, and guide you through creating custom configurations using ‘@Conditional’.
Spring Boot Auto Configuration Unveiled:
Imagine you're starting a new project using Spring Boot. You've heard it's great for building Java applications, but you're not sure where to begin with all the setup. That's where Spring Boot's Auto Configuration comes into play. It’s like having a helpful assistant who reads your mind and sets up things for you.
Understanding Spring Boot Auto Configuration:
Spring Boot Auto Configuration is a feature that aims to minimize the configuration burden on developers by automating the setup of various components within a Spring application. When a Spring Boot application starts up, it scans the classpath for specific libraries and components, and based on the presence of these components, it automatically configures beans, services, and other resources. This eliminates the need for developers to manually configure these components, resulting in a faster and more streamlined development process.
How Auto Configuration Helps:
- · Saves Time: With Auto Configuration, you can jump straight into coding your app without spending too much time on repetitive setup tasks.
- · Less Configuration: You don't need to tell Spring Boot every little detail about your app; it's smart enough to figure things out on its own.
- · No More Guesswork: Spring Boot takes care of complex setups, so you don't need to guess which libraries to include or which beans to configure.
Real-world Examples of Auto Configuration:
Let's look at some familiar examples that Spring Boot's Auto Configuration handles effortlessly:
- Ø Database Connection: You want to connect your app to a database. Spring Boot notices the database driver in your project and sets up the database connection without you writing a ton of code.
Example: If you include the H2 database dependency in your project, Spring Boot automatically configures a DataSource bean and sets up a connection. - Ø Building Web Apps: If you're creating a web application, Spring Boot automatically configures the necessary components, like setting up a web server and handling HTTP requests.
Example: Including the Spring Web dependency leads to automatic setup of a web server, and you can start building your web endpoints right away. - Ø Data Management: When using technologies like JPA for managing data, Spring Boot saves you from manually setting up an EntityManager by doing it for you.
Example: With JPA dependencies added, Spring Boot sets up an EntityManagerFactory and transaction management for you to work with databases.
Purposes of Spring Boot Auto Configuration:
- · Developer Productivity: Auto Configuration saves developers' time and effort by handling repetitive configuration tasks, allowing them to focus on implementing business logic.
- · Convention over Configuration: Spring Boot follows the "convention over configuration" principle, where sensible defaults are provided based on the context, reducing the need for explicit configuration.
- · Consistency: Auto Configuration ensures that different projects follow similar setups, promoting a consistent development and deployment environment.
Advantages of Spring Boot Auto Configuration:
- · Rapid Development: Developers can create functional applications quickly by leveraging the pre-configured components provided by Auto Configuration.
- · Reduced Configuration Errors: Since Spring Boot handles configuration, the chances of making configuration mistakes are significantly reduced.
- · Easy Upgrades: When libraries or dependencies are updated, Spring Boot's Auto Configuration helps ensure that the necessary adjustments are made automatically.
Disadvantages of Spring Boot Auto Configuration:
- · Overhead: Auto Configuration can lead to the inclusion of unnecessary components, increasing the application's memory footprint.
- · Less Control: Developers might have limited control over the configuration details, making it challenging to fine-tune certain aspects.
- · Complexity: Understanding the inner workings of Auto Configuration and troubleshooting issues can be complex, especially in larger applications.
Crafting Your Own Auto Configuration with @Conditional:
Feeling adventurous? Spring Boot allows you to create your own Auto Configuration using the @Conditional annotation. This is like giving your assistant some specific instructions.
Example:
Let's say you want to add special caching to your app only when a certain feature is enabled. You can create an Auto Configuration class like this:
@Configuration
@Conditional(EnableCustomCachingCondition.class)
public class CustomCachingAutoConfiguration {
// Your custom caching setup
}
@Configuration
@Conditional(EnableCustomCachingCondition.class)
public class CustomCachingAutoConfiguration {
// Your custom caching setup
}
Here, EnableCustomCachingCondition is a custom check that ensures the caching setup only happens when the right conditions are met.
The Journey Continues:
Congratulations, you've taken your first steps into the world of Spring Boot Auto Configuration! By now, you understand how this clever feature saves you time and helps you build amazing applications without drowning in setup details. Remember, while Auto Configuration is incredibly handy, knowing when and how to customize it will make you a true Spring Boot maestro. So, keep exploring, experimenting, and crafting wonderful applications with Spring Boot's Auto Configuration magic. Happy coding!
Spring Boot Auto Configuration and Creating Custom Auto Configuration with @Conditional

August 23, 2023