Spring Boot Microservice API Development with Feign Client + Eureka + Spring Cloud Loadbalancer
Java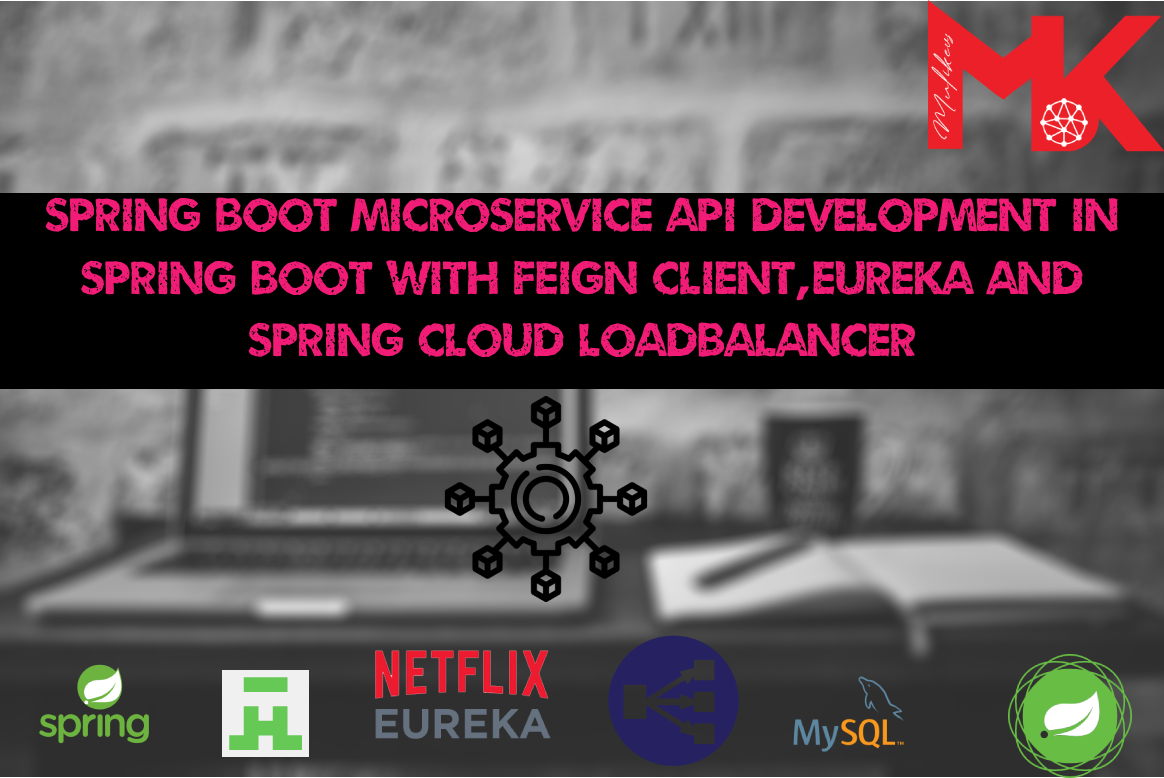
Spring Boot Microservice API Development with Feign Client + Eureka + Spring Cloud Loadbalancer
Microservices are a popular architectural pattern for building scalable and resilient applications. One of the challenges of microservices is how to develop APIs that allow different microservices to communicate with each other.
Spring Boot is a popular framework for developing microservices. It provides several features that make it easy to develop and deploy microservices, including:
- · Autoconfiguration: Spring Boot automatically configures many of the dependencies that are needed to run a microservice.
- · Starters: Spring Boot provides starters that make it easy to add common dependencies to a microservice, such as web servers, databases, and messaging.
- · Actuator: Spring Boot provides an actuator that allows you to monitor and manage your microservices.
Feign Client is a declarative HTTP client that makes it easy to interact with RESTful APIs. It is a great way to decouple your code from the underlying implementation of the API.
Eureka is a service discovery framework that allows you to register and discover microservices. This makes it easy to scale your microservices architecture by adding or removing microservices without having to change your code.
Spring Cloud Loadbalancer is a library that provides load balancing for Spring Boot applications. It makes it easy to distribute traffic across multiple instances of a microservice, which improves performance and availability.
Advantages of using Feign Client, Eureka, and Spring Cloud Loadbalancer
There are several advantages to using Feign Client, Eurka, and Spring Cloud Loadbalancer in a microservices architecture:
- · Decoupling: Feign Client decouples your code from the underlying implementation of the API, which makes it easier to change the API without having to change your code.
- · Discovery: Eureka makes it easy to discover microservices, which makes it easy to build applications that use multiple microservices.
- · Load balancing: Spring Cloud Loadbalancer makes it easy to distribute traffic across multiple instances of a microservice, which improves performance and availability.
- · Fault tolerance: Feign Client can automatically retry requests that fail, which makes your microservices more fault tolerant.
Disadvantages of using Feign Client, Eureka, and Spring Cloud Loadbalancer
There are also some disadvantages to using Feign Client, Eureka, and Spring Cloud Loadbalancer:
- · Complexity: Feign Client can be complex to use, especially for more complex APIs.
- · Performance: Feign Client can add some overhead, which can impact performance.
- · Security: Feign Client does not provide any security features out of the box, so you need to implement security yourself.
Conclusion
Feign Client, Eureka, and Spring Cloud Loadbalancer are three powerful tools that can be used to develop APIs in a microservices architecture. They offer a number of advantages, but they also have some disadvantages.
The best tool for your project will depend on your specific needs and requirements. If you are looking for a way to decouple your code from the underlying implementation of the API, Eureka, and Spring Cloud Loadbalancer are a good option. If you are looking for a way to improve performance and availability, Spring Cloud Loadbalancer is a good option.
Spring Boot Microservice Example
Here is a sample Spring Boot microservice project that uses Feign Client, Eureka, and Spring Cloud Loadbalancer:
git clone https://github.com/mulikevs/microservices.git
cd microservices
This project has four microservices currently (Might have more):
- · Discovery-service: This microservice provides a EUREKA Server.
- · Address Service: This microservice provides a REST API for managing addresses.
- · Employee Service: This microservice provides a REST API for managing employees.
- · Customer Service: This microservice provides a REST API for managing customers.
All The above microservices are using one MySql Database(Not Recommended in a Microservice Architecture), So make sure you have changed the configurations for the Database in all the application.properties File.
Sample File For the employee.service
spring.datasource.driver-class-name =com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/sitemapdevmicro
spring.datasource.username=root
spring.datasource.password=mysql
spring.jpa.show-sql=true
spring.jpa.generate-ddl=true
spring.jpa.hibernate.ddl-auto=validate
spring.jpa.database-platform=org.hibernate.dialect.MySQL5InnoDBDialect
spring.jpa.properties.hibernate.formart_sql=true
spring.application.name =employee-app
server.port=8090
server.servlet.context-path=/employee-app/api
management.endpoints.web.exposure.include=*
management.info.env.enabled=true
info.app.name=Employee App
info.app.version=1.0.0
#Below is for testing, to be removed
addressservice.base.url= http://127.0.0.1:8091/address-app/api
spring.datasource.driver-class-name =com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/sitemapdevmicro
spring.datasource.username=root
spring.datasource.password=mysql
spring.jpa.show-sql=true
spring.jpa.generate-ddl=true
spring.jpa.hibernate.ddl-auto=validate
spring.jpa.database-platform=org.hibernate.dialect.MySQL5InnoDBDialect
spring.jpa.properties.hibernate.formart_sql=true
spring.application.name =employee-app
server.port=8090
server.servlet.context-path=/employee-app/api
management.endpoints.web.exposure.include=*
management.info.env.enabled=true
info.app.name=Employee App
info.app.version=1.0.0
#Below is for testing, to be removed
addressservice.base.url= http://127.0.0.1:8091/address-app/api
Run Your Service Discovery, Address, Customer, and Employee Microservices
Now run your Service Discovery, Address, Customer, and Employee Microservices. If everything goes well then you may see the following screen in your console. Please refer to the below image.
Spring Eureka Dashboard:
Test Your Endpoints in Postman
The Employee Service uses Feign Client to call the Address Service. This allows the Employee Service to decouple its code from the underlying implementation of the Address Service.
The Employee Service and Address Service are both registered with Eureka. This allows them to discover each other and communicate with each other.
Spring Cloud Loadbalancer is used to distribute traffic across multiple instances of the Employee Service. This ensures that the Employee Service is always available.
Conclusion
This blog article has introduced Feign Client, Eureka, and Spring Cloud Loadbalancer. These are three powerful tools that can be used to develop APIs in a microservices architecture. They offer several advantages, but they also have some disadvantages.
The best tool for your project will depend on your specific needs and requirements. I hope this blog article was helpful. Please let me know if you have any questions.
You Can Download the above code from GitHub
Spring Boot Microservice API Development with Feign Client + Eureka + Spring Cloud Loadbalancer

August 03, 2023