Spring Boot & The Database Dance: From Relational to NoSQL.
Java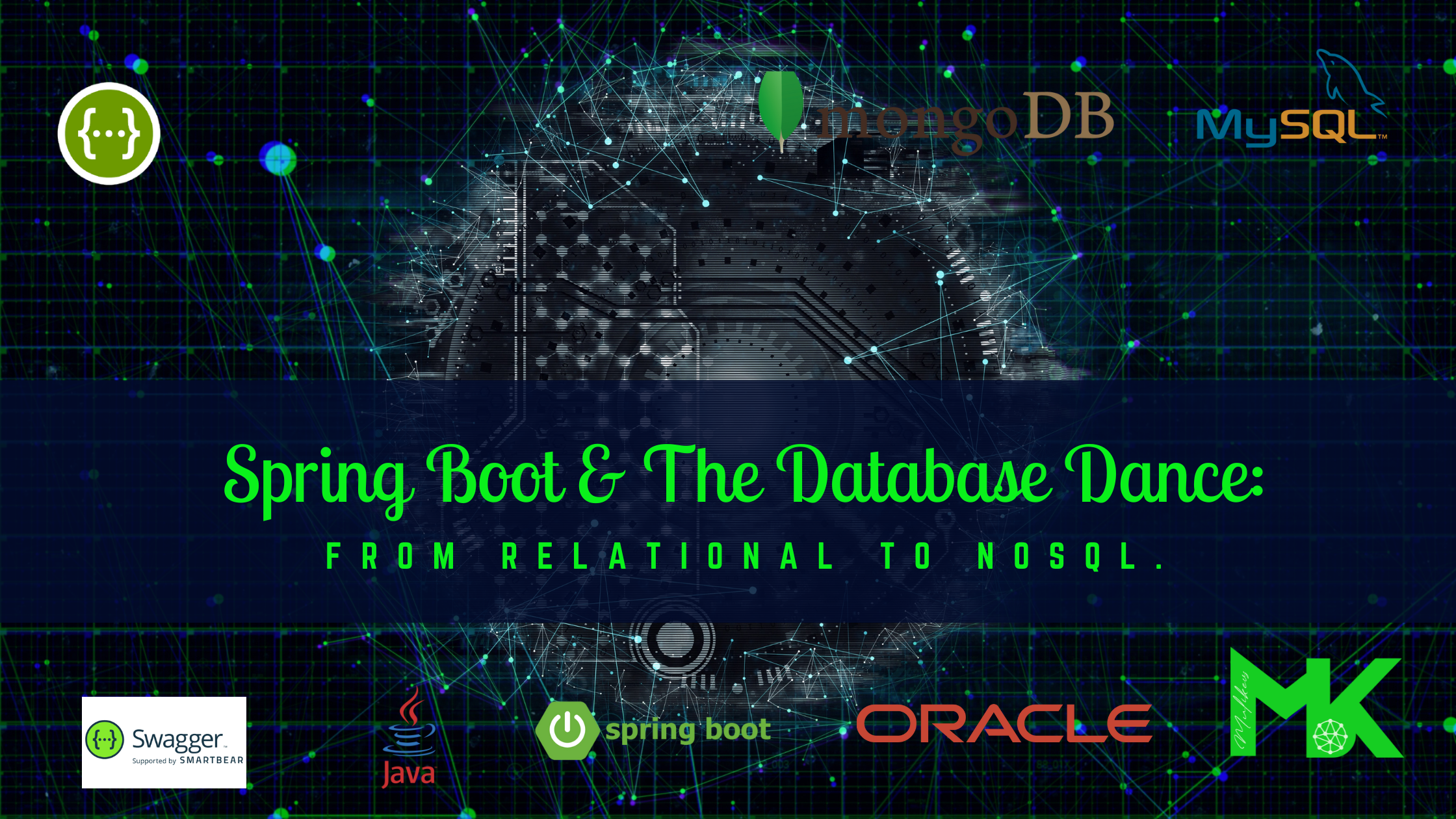
Spring Boot & The Database Dance: From Relational to NoSQL.
Spring Boot shines in simplifying application development, and choosing the right database is crucial for efficient data storage and retrieval. This post explores popular databases compatible with Spring Boot, along with crafting SQL queries for relational and NoSQL databases. We'll also dive into factors to consider when making your database selection.
Relational Databases: Structured and Familiar
Relational databases, like MySQL, PostgreSQL, and Oracle, store data in well-defined tables with rows and columns. They excel in structured data and complex queries using SQL (Structured Query Language).
Normal to Complex SQL Queries:
· Simple Select: Retrieve specific columns from a table:
SELECT name, email FROM users;
· Conditional Select: Filter data based on conditions:
SELECT * FROM products WHERE price > 100;
· Joins: Combine data from multiple tables:
SELECT users.name, orders.product_idFROM users INNER JOIN orders ON users.id = orders.user_id;
· Aggregates: Perform calculations on groups of data:
SELECT COUNT(*), category FROM products GROUP BY category;
Spring Boot with Relational Databases:
Spring Data JPA (Java Persistence API) provides a powerful abstraction layer for interacting with relational databases using familiar Java objects. Spring Boot auto-configuration simplifies connecting to various databases.
Here's a complete code snippet demonstrating CRUD operations with JPA:
@Entitypublic class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String name; private String email; // Getters and Setters}
@Repositorypublic interface UserRepository extends JpaRepository<User, Long> { Optional<User> findByName(String name);}
@Controllerpublic class UserController {
@Autowired private UserRepository userRepository;
@PostMapping("/users") public User createUser(@RequestBody User user) { return userRepository.save(user); }
// Similar methods for Read, Update, and Delete}
NoSQL Databases: Flexible and Scalable
NoSQL databases, like MongoDB, Cassandra, and Couchbase, offer flexibility for storing data in various formats (documents, key-value pairs, etc.). They excel in handling large, unstructured datasets and scale easily.
NoSQL Querying:
NoSQL databases typically have their own query languages, often document oriented. Here's an example using MongoDB's MQL (MongoDB Query Language):
// Find all users with email "john.doe@example.com"db.users.find({ email: "john.doe@example.com" });
// Find users with age greater than 30db.users.find({ age: { $gt: 30 } });
Spring Boot with NoSQL Databases:
Spring Data provides integrations for various NoSQL databases. For example, Spring Data MongoDB allows interacting with MongoDB collections using familiar Java object-document mapping.
Here's a simplified example of finding a user by email in Spring Boot with MongoDB:
@Document(collection = "users")public class User { @Id private String id; private String name; private String email; // Getters and Setters}
@Repositorypublic interface UserRepository extends MongoRepository<User, String> { Optional<User> findByEmail(String email);}
@Controllerpublic class UserController {
@Autowired private UserRepository userRepository;
@GetMapping("/users/{email}") public User getUserByEmail(@PathVariable String email) { return userRepository.findByEmail(email); }}
Choosing the Right Database: It Depends
The choice between relational and NoSQL databases hinges on several factors:
- Data Structure: Relational databases are ideal for structured data with well-defined relationships between tables. NoSQL databases cater to flexible data models that may evolve over time.
- Access Patterns: If your application requires complex queries involving joins and aggregations, relational databases might be a better fit. NoSQL databases shine in applications with frequent inserts, updates and simple queries that prioritize quick data retrieval over complex joins.
- Scalability: Relational databases can struggle with massive datasets, while NoSQL databases excel at scaling horizontally.
- Consistency: Relational databases guarantee ACID (Atomicity, Consistency, Isolation, Durability) properties, ensuring data integrity. NoSQL databases may offer eventual consistency, where data updates take time to reflect across all replicas.
- Expertise: Consider your team's experience. Relational databases have a longer history and wider skillset availability.
Spring Boot & The Database Dance: From Relational to NoSQL.

March 18, 2024